Can you improve an API Speed ?
can we 10x Our API Performance ? practical ways to improve API performance
Special thanks to Osama Zinhom for sharing these questions. This post, along with the others in the series, is based on a conversation with ChatGPT about these topics. You can view the original questions here: Osama's LinkedIn Post.
APIs (Application Programming Interfaces) are like messengers that allow apps to talk to each other. If your API is slow, the app that depends on it will also be slow, and this can frustrate users. Imagine you are using a food delivery app, and when you search for nearby restaurants, it takes 10 seconds to show results. Even though the app eventually gets the data, the long wait time would likely make you feel impatient or cause you to stop using the app.
In short, speed directly affects how users perceive the quality of the app.
Techniques to Improve API Speed
Here are some common methods to make an API faster:
1. Reduce Payload Size
The data sent between the server and the client should be as small as possible. If you are sending unnecessary data, it will take longer to transfer and process.
Example: If the API is sending this JSON response:
{ "id": 123, "name": "John Doe", "age": 30, "address": "123 Main St", "phone": "123-456-7890", "extraInfo": "Some unnecessary details" }
If the client doesn’t need extraInfo, you should remove it to make the payload smaller:
{ "id": 123, "name": "John Doe", "age": 30, "address": "123 Main St", "phone": "123-456-7890" }
2. Caching
Instead of fetching data from the server every time, you can store (cache) it temporarily. If the same data is requested again, you return it from the cache, which is much faster.
Use Case: In a weather app, the forecast for a city might not change every second. You can cache the forecast for a few minutes, so users get instant results when they request it within that time frame.
3. Use Compression
Compressing the data before sending it can reduce its size, making it faster to transfer.
Example: You can enable Gzip compression in your API so that large responses are compressed:
Content-Encoding: gzip
4. Database Optimization
APIs often pull data from a database. If the database queries are slow, the API will be slow as well. You can optimize the database by indexing frequently searched columns or by using more efficient queries.
Example: If your API is slow because it fetches a lot of data, you can improve the query to get only the needed rows:
SELECT name, age FROM users WHERE age > 30;
Instead of:
SELECT * FROM users;
5. Asynchronous Processing
If the API has to do heavy tasks (like processing a large amount of data), you can make it run in the background, so the API returns quickly while the task continues separately.
Use Case: In an e-commerce app, if a user places an order, instead of waiting for the order to be fully processed (checking inventory, calculating shipping, etc.), the API can immediately confirm the order and send the user a notification once processing is done.
6. Rate Limiting
By limiting the number of requests a user can make in a certain time frame, you prevent the API from being overwhelmed, which can slow down the response time.
Use Case: If a user tries to refresh a dashboard too many times in a minute, the API can return a message like "Too many requests" and block further requests until the limit resets.
Code Example: Optimized API with Caching and Reduced Payload
Here’s a basic example of an API using Node.js and Express, where we reduce the payload and add caching.
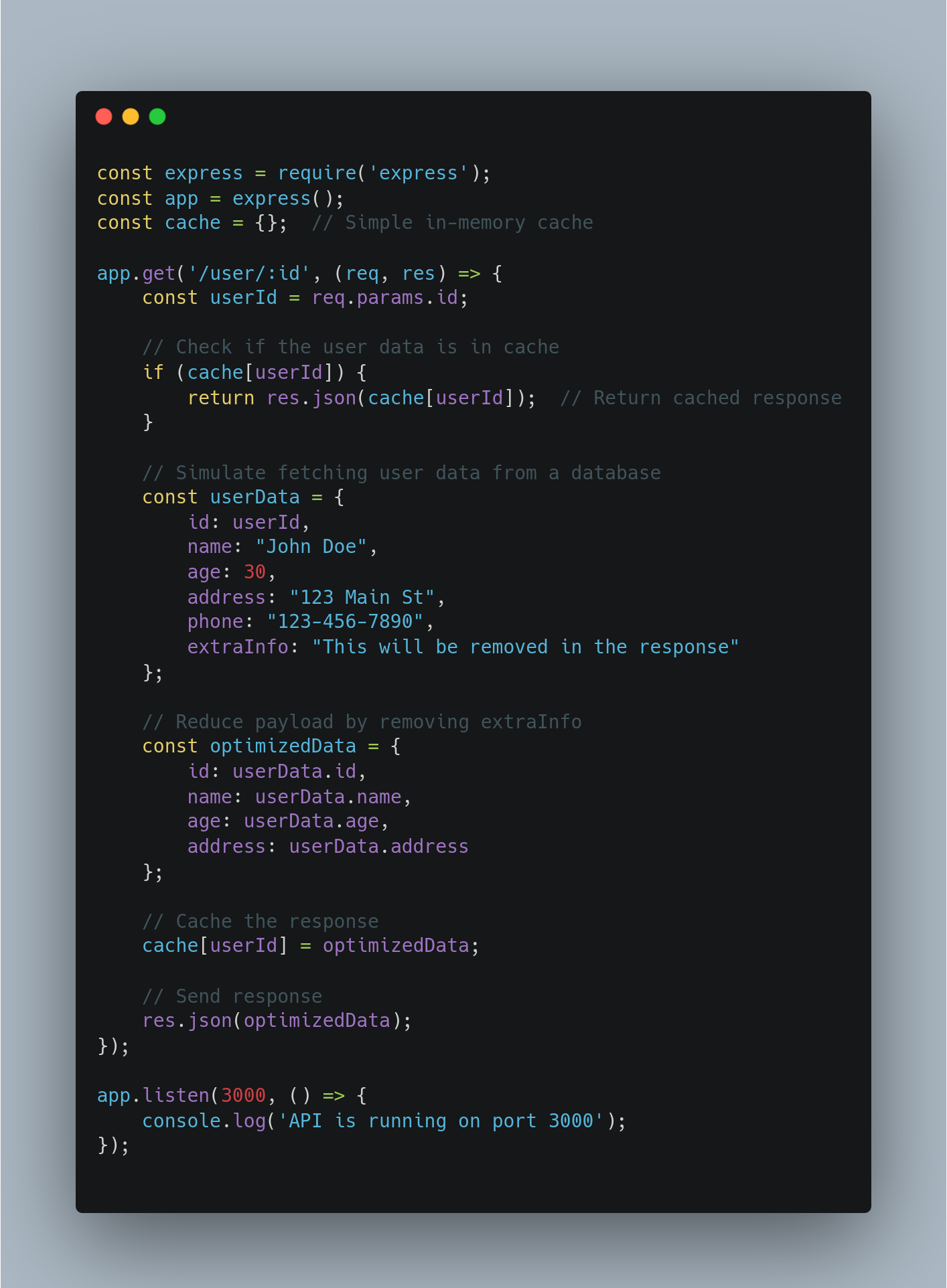
Summary:
Extra Reads and Resources: