"Monty: Stacks on Stacks: A LIFO & FIFO Love Story PART 2"
ALXSE oriented guide - 0x19. C - Stacks, Queues - LIFO, FIFO - Monty 1st.
In the realm of data structures,
a choice must be made,
Between Stack and Queue,
where paths diverge and sway.
Stack, the mischievous, embraces the last in, first out,
While Queue, patient and orderly, follows first in, first out.
How do I use extern to share variables between source files in C?
In C, the extern
keyword is used to declare a variable that is defined in a different source file. This allows multiple source files to share the same variable, and is useful when you want to break up a large program into smaller, more manageable modules.
Here's an example of how to use extern
to share a variable between two source files:
In file1.c
:
int shared_variable = 10;
In file2.c
:
extern int shared_variable;
//
use the shared variable in some way
void do_something() {
printf("The value of the shared variable is %d\n", shared_variable);
}
Important note :
To compile the code correctly, you will need to first compile the source file that contains the definition of the shared
variable, and then compile the source file that contains the main
function and the extern
declaration.
Here's an example of how you could compile the code using the gcc
compiler on a Unix-like system:
gcc -c ext1.c
gcc -o program ext2.c ext1.o
So What is a global variable and how it differs from extern ?
A global variable is a variable that is declared outside of any function in a C program. This means that the variable can be accessed from any function in the same source file. Here's an example:
// global variable
int global_variable = 10;
void function1() {
//
accessing the global variable
printf("The value of the global variable is %d\n", global_variable);
}
void function2() {
//
modifying the global variable
global_variable = 20;
}
In this example, global_variable
is a global variable that can be accessed and modified from any function in the same source file.
On the other hand, extern
is used to declare a variable that is defined in a different source file. This allows multiple source files to share the same variable.
So, the main difference between a global variable and an
extern
variable is that a global variable is defined in the same source file and can be accessed from any function in that file, while anextern
variable is defined in a different source file and can be accessed from any function in that file as well as any other file that includes its declaration.
It's worth noting that the use of global variables can make a program more difficult to understand and maintain, especially in larger projects. It's often better to use local variables and pass them as arguments to functions, or to use other techniques such as encapsulation and data hiding to manage program state.
What is a stack, and when to use it ?
In general, a stack can be used whenever you need to store a collection of items in a specific order and retrieve them in reverse order. Some other examples of applications of stacks include backtracking algorithms, undo/redo functionality in applications, and browser history.
What is a queue, and when to use it ?
a queue is a data structure that stores and retrieves data based on the first-in, first-out (FIFO) principle. This means that the first item added to the queue is the first one to be removed, and the last item added is the last one to be removed.
A queue is typically implemented as an abstract data type, meaning that it is defined by its behavior and the operations that can be performed on it. The two main operations that can be performed on a queue are:
Enqueue: Add an item to the back of the queue.
Dequeue: Remove the item from the front of the queue.
In addition to these basic operations, a queue may also support other operations such as peek (accessing the front item without removing it), size (counting the number of items in the queue), and isEmpty (checking if the queue is empty).
One common use of a queue is in task scheduling. When multiple tasks need to be processed, they can be added to a queue in the order they were received, and then processed in the same order. This ensures that each task is processed fairly and in the order it was received.
Another use of a queue is in message passing systems. When messages are sent between processes or threads, they can be added to a queue and processed in the order they were received.
Note that FIFO (First-In-First-Out): the first element inserted is the first one to be removed. This means that the order of insertion is not preserved, as the most recently added element will be the last one to be removed. Therefore, FIFO reverses the order of insertion.
The Monty language
The Monty language is a scripting language that is compiled into Monty byte codes. It shares some similarities with Python in terms of its compilation process. The purpose of the Monty language is to provide a unique stack and specific instructions to manipulate it.
The main objective of the Monty project is to develop an interpreter specifically designed to execute Monty ByteCodes files. These files typically have a .m extension, although it is not mandated by the language specification.
For example, a line in a Monty byte code file may look like this:
push 10
//values are printed to the terminal using the pall instruction.
pall
In this case, "push" is the opcode, and "10" is the argument. The interpreter would interpret this instruction as pushing the value 10 onto the stack.
The Monty language and its byte code files are commonly used in the industry, and the .m extension is widely adopted.
Overview :Error handling
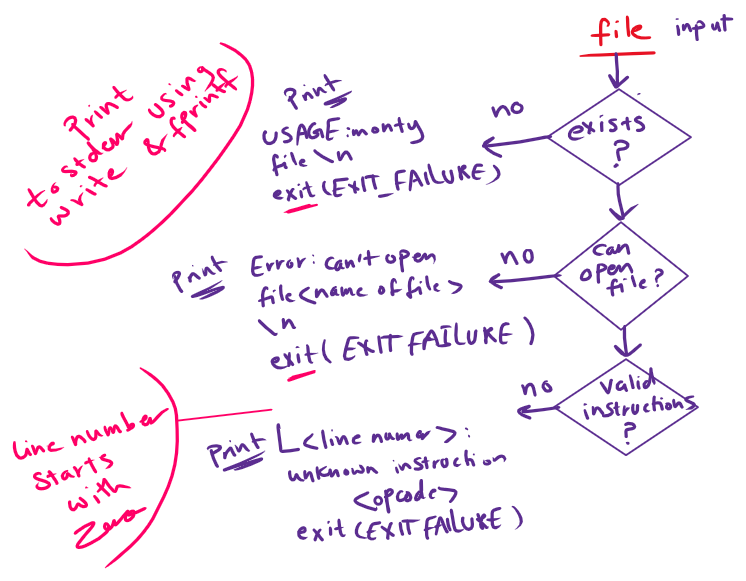
USAGE: monty file
, followed by a new line, and exit with the status EXIT_FAILURE
Program Execution :
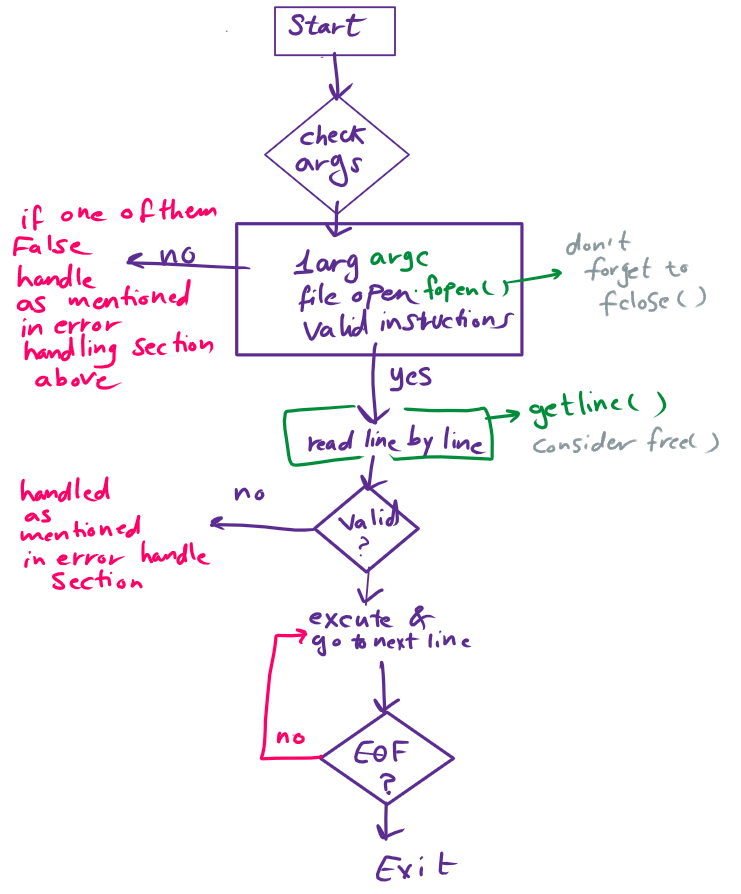
Read file line by line
a. If instruction is valid, execute it and proceed to next line
b. If instruction is invalid, print error message with line number and exit with status EXIT_FAILURE
c. If end of file is reached or an error occurs, exit program
How To Use a Test Suite:
To use the any test_suite
in your program, you need to follow these steps:
Download the
test_suite
directory, which contains the test suite files for themonty_project
.Place the
alx_test_suite
directory in the same location as yourmonty_project
directory. Make sure the directory structure looks like this:
Open your terminal or command prompt and navigate to the root directory of your project (the directory that contains both
monty_project
andtest_suite
).Run the test suite by executing the test files one by one using a shell command. For example, to run the first test file (
0-no_argument.sh
), you can use the following command:
$ cd monty_project
$ sh test_suite/0-no_argument.sh
Repeat the above step for all the test files in the
test_suite
directory. You can execute each test file individually or create a script that runs all the test files at once.Observe the output of each test file. The test suite will run various tests against your
monty_project
program and provide the corresponding output for each test case. It will help you verify if your program is functioning correctly.Assure that you first compile ,executable name is monty and all in the same directory.
Stay tuned it’s an updating content..
Resources :
warning: implicit declaration of function 'getline'
check part 1 :