How to open a file:
The open()
function returns a file object that you can use to read, write, or manipulate the contents of the file. Here's the basic syntax for 2 ways of opening a file in Python:
my_file = open("hello.txt", "r") # first way
my_file.close()
with open("hello.txt", "r"): # second way
print("File is open")
with open statement versus open():
The with
statement in Python provides a convenient way to automatically close files after they're used, even if an error occurs in the code.
with open('example.txt', 'r') as file:
# perform operations on file object
Using the
with
statement is generally considered a best practice when working with files in Python, as it ensures that files are always properly closed, even if an error occurs in the code.On the other hand, using the
open()
function without thewith
statement requires you to explicitly close the file using theclose()
method on the file object, which can be easy to forget and can lead to resource leaks if not done properly.
How to write text in a file:
It's important to always close the file when you're done writing to it, as this ensures that the data is properly flushed to disk and the file is released from memory.
Note that if the file already exists, opening it in write mode will overwrite its contents. If you want to append text to an existing file instead of overwriting it, you can open the file in append mode by passing the 'a'
argument to the open()
function:
file = open('example.txt', '
a')
file.write('This is some additional text.\n')
file.close()
Error to avoid:
FileExistsError
. This error occurs when you try to open a file that already exists. The error message will be something like this:
FileExistsError: [Errno 17] File exists: 'hello.txt'
The error occurs when an open()
statement opens the file in read mode. another open()
statement also tries to open the file in read mode. This will cause an error because the file is already open.
How to read the full content of a file:
We can use the read()
method on the file object to read the full contents of the file into a string variable like this:
Note that the read()
method reads the full contents of the file into memory as a string, so it may not be suitable for very large files that won't fit into memory. In that case, you may want to read the file line by line using a loop and the readline()
method on the file object, or use a more memory-efficient approach such as using a generator function and the yield
keyword to read the file in chunks.
How to read a file line by line ?
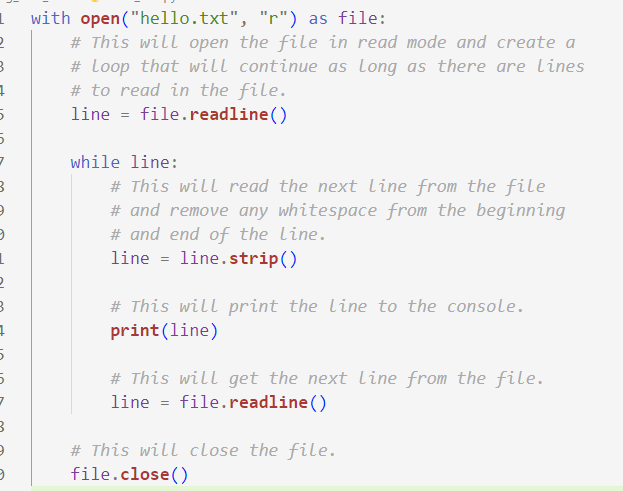
readline()
method, and then read subsequent lines inside the loop using the same method. This is because the first call to readline()
is needed to initialize the line
variable with the first line of the file.Note: Without this second call to readline()
, we would be stuck processing the first line of the file over and over again in an infinite loop.
How to move the cursor in a file: seek()
In Python, you can use the seek()
method on a file object to move the cursor to a specific position in the file. The seek()
method takes a byte offset as its argument, which specifies the position in the file to move the cursor to. Here's an example:
We can use the
read(n)
method instead of theread()
method. Theread(n)
method will read the nextn
bytes from the file. In this case, you would need to use theread(-1)
method to read the contents of the file from the current cursor position to the end of the file.
What is JSON ?
JSON stands for "JavaScript Object Notation". It is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. JSON is often used for data exchange between web servers and web clients, as well as between different parts of a web application.
JSON is based on a subset of the JavaScript
, It consists of a collection of name/value pairs, where the names are strings and the values can be strings, numbers, booleans, arrays, or other JSON objects. Here's an example of a simple JSON object:
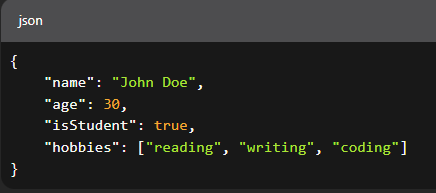
json
module to encode Python objects as JSON
strings, and decode JSON strings back into Python objects.What is serialization ?
Serialization is the process of converting data structures or objects into a format that can be stored or transmitted, and later reconstructed into their original form. The serialized data can be stored in a file, database, or sent over a network, and can be reconstructed later when needed.
In Python, the pickle
module provides a way to serialize and deserialize Python objects. The pickle
module can convert Python objects into a binary format that can be stored or transmitted, and then deserialize them back into their original form. Here's an example:
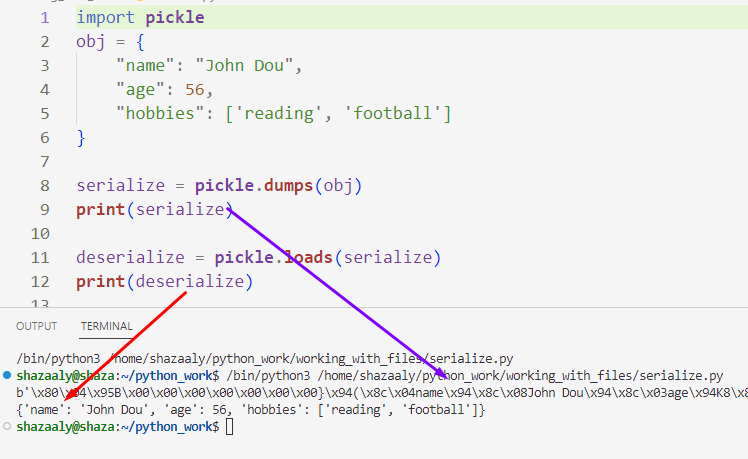
dumps()
method of the pickle
module to serialize the data
object into a binary format, which we store in a variable called serialized_data
.We can later deserialize the serialized_data
back into a Python object using the loads()
method of the pickle
How to convert a Python data structure to a JSON string?
In Python, you can use the built-in json
module to convert a Python data structure, such as a dictionary or a list, to a JSON string. The json
module provides two main methods for encoding Python objects as JSON strings: json.dumps()
and json.dump()
.
Here's an example using the json.dumps()
method to convert a Python dictionary to a JSON string:
json(dumps)vs json(dump)
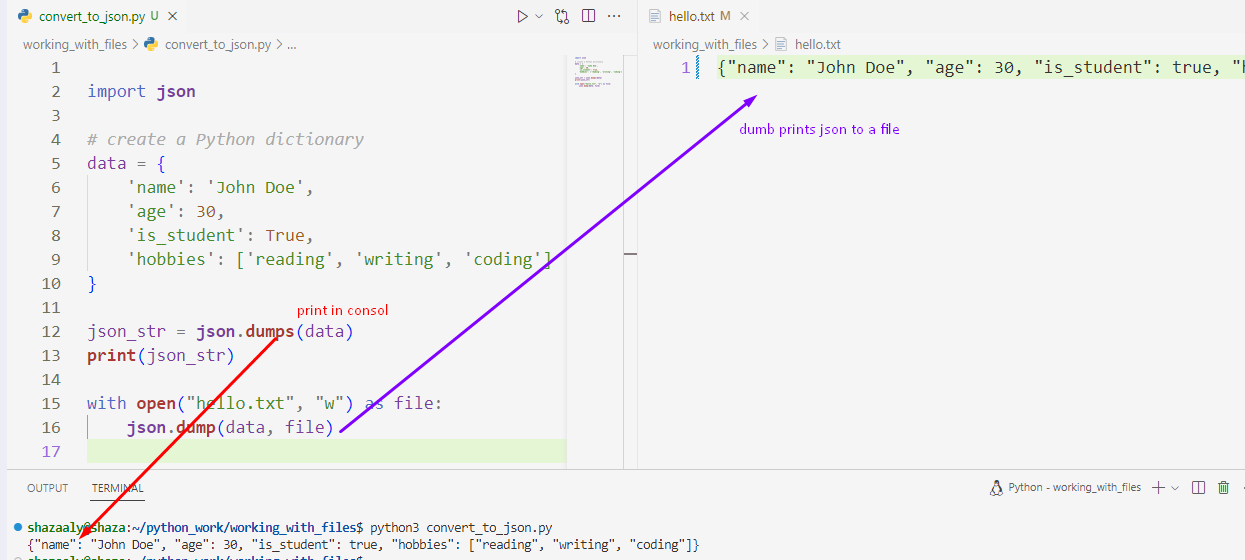
json.dump()
method to write the data
dictionary directly to a file named "data.json" in JSON format.How to convert a JSON string to a Python data structure?
In Python, you can use the built-in json
module to convert a JSON string to a Python data structure, such as a dictionary or a list. The json
module provides two main methods for decoding JSON strings: json.loads()
and json.load()
.
Here's an example using the json.loads()
method to convert a JSON string to a Python dictionary:
The json.loads()
and json.load()
methods in Python's json
module are used to convert a JSON string to a Python object and a JSON file to a Python object respectively.
json.loads()
is used to load a JSON string into a Python object. It takes a single string argument that contains a valid JSON data and returns a corresponding Python object. Here's an example:
import json
# JSON string
json_string = '{"name": "John Doe", "age": 30, "is_student": true, "hobbies": ["reading", "writing", "coding"]}'
# convert JSON string to Python object
data = json.loads(json_string)
# print the Python object
print(data)
json.load()
is used to load a JSON file into a Python object. It takes a single file object argument that contains a valid JSON data and returns a corresponding Python object. Here's an example:
import json
# read JSON file
with open('data.json', 'r') as f:
# convert JSON file to Python object
data = json.load(f)
# print the Python object
print(data)
The difference between
json.loads()
andjson.load()
is thatjson.loads()
loads a JSON string, whereasjson.load()
loads a JSON file. In both cases, the resulting Python object is the same.It's worth noting that
json.load()
can only be used with a file object, whereasjson.loads()
can be used with a string containing the JSON data.
More to understand differences between pickle and json methods :