Unit Testing for Command-Line Interfaces (CMD) in Python
AirBnB Clone Console - unittest - mock -patch - console.py
I'll walk you through the basics of creating unit tests for a custom command-line interface (CLI) application in Python using the unittest
framework. We'll cover how to simulate user input, capture console output, and make assertions to verify the behaviour of your CLI commands.
The idea is to capture the output of the custom command prompt messages and then assert that the captured output matches your expectations.
Suppose you have a Python script cmd.py
that includes a function get_custom_message()
that prints a custom message to the command prompt using the print()
function. You want to test this behavior without actually printing to the console during your unit tests.
Import Necessary Modules: In your test file (
test_cmd.py
), import the required modules, includingunittest
andunittest.mock
:from unittest.mock import patch
Note:
Mocks are imitation objects used for testing, and patching is the technique of temporarily replacing parts of your code with mock objects to control behavior and interactions during testing. This helps you isolate and test specific units of your code more effectively.
Here we need patch()
function from the unittest.mock
module to temporarily replace the standard output stream (sys.stdout
) with a StringIO
object.
"sys.stdout"
: This is the target we want to patch (replace). In this case, we're targeting the standard output stream, which is where theprint()
function writes its output.
new=StringIO()
: This specifies what the target should be replaced with. We're creating a newStringIO
object, which is an in-memory file-like object that we can use to capture the output that would have been sent to the standard output.
Example:
Suppose you have the following Python script named greeting.py
:
And you want to test the print_greeting()
function without actually printing to the console.
note : from io import StringIO
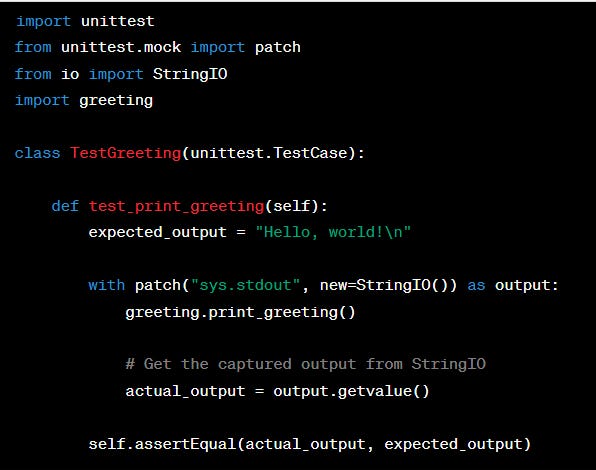
print_greeting()
function without actually displaying it on the console. This way, you can test the behavior of the function in isolation.This approach is useful for testing CLI commands or functions that interact with the command prompt and have side effects like printing to the console. By using patch
to capture and suppress the console output, you can focus on testing the actual behavior of the command without cluttering the test results with unnecessary console output.
More Examples:
Capturing and Checking Output
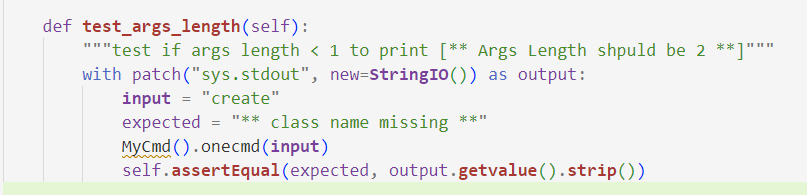
output.getvalue()
function is commonly used with the StringIO
object to retrieve the content stored within it. This is particularly useful when capturing console output during testingMyCMD().onecmd(input)
:
MyCMD()
refers to an instance of theMyCMD
class. It appears thatMyCMD
is a class representing a command-line interface (CLI) command handler..onecmd(input)
is a method of theCMD
class (or a class derived from it) that is used to execute a command specified by the user. Theinput
variable contains the command to be executed.
By calling
.onecmd(input)
, you're simulating the execution of a command within the CLI. The command's output (if any) will be generated during this execution.
The output generated by executing the command is usually printed to the console. In the context of your unit test, the
StringIO
object from thepatch
is capturing this output, allowing you to later check and assert against it.
output.getvalue()
:
output
refers to theStringIO
object that you created within thepatch
context. It's capturing the console output that was generated during the execution of the command..getvalue()
is a method provided by theStringIO
class. It retrieves the contents of theStringIO
buffer, which in this case contains the captured console output.In the context of your test,
output.getvalue()
allows you to obtain the captured output so that you can compare it against your expected output for assertions.
Note : Don’t forget to import needed modules :
import unittest
from unittest.mock import patch
from io import StringIO
from mycmd_file import MyCMD #
according to your filename and module name
More on Airbnb and Unit tests : ..